How to Create A REST API With JSON Server ?
Last Updated :
15 May, 2024
Setting up a RESTful API using JSON Server, a lightweight and easy-to-use tool for quickly prototyping and mocking APIs. JSON Server allows you to create a fully functional REST API with CRUD operations (Create, Read, Update, Delete) using a simple JSON file as a data source.
Approach
- First, Create a JSON file that represents your data model. This JSON file will serve as your database.
- Run JSON Server and point it to your JSON file using the command npx json-server --watch users.json.
- Create four different JavaScript files to perform the operations like Create, Read, Update, Delete.
- To Send a GET Request use the command in the terminal node get_request.js that returns a list of all users stored on the server, Send a POST request to create a new user, this will create a new user with the provided data.
- Similarly, Send a PUT request to update an existing user. This will update the user with the provided data and for DELETE Request, The endpoint deletes the user with the specified id from the server. After deletion, the user will no longer exist in the database.
Steps to create a REST API with JSON Server
Run the below command to Create a package.json file:
npm init -y
Run the below command to Install the JSON Server globally using npm:
npm i -g json-server
Run the below command to Install Axios:
npm install axios
Project Structure:
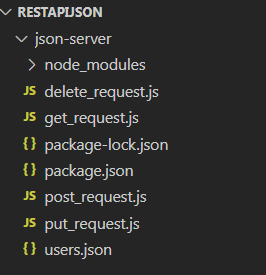
Example: The example below shows the JSON file that represents your data model.
JavaScript
{
"users": [
{
"id": "1",
"first_name": "Ashish",
"last_name": "Regmi",
"email": "ashish@gmail.com"
},
{
"id": "2",
"first_name": "Anshu",
"last_name": "abc",
"email": "anshu@gmail.com"
},
{
"id": "3",
"first_name": "Shreya",
"last_name": "def",
"email": "shreya@gmail.com"
},
{
"id": "4",
"first_name": "John",
"last_name": "aaa",
"email": "John@yahoo.com"
},
{
"first_name": "Geeks For",
"last_name": "Geeks",
"email": "gfg@gmail.com",
"id": "5"
}
]
}
Run the below command to start JSON Server and point it to your JSON file:
npm json-server --watch users.json
GET Request Returns a List of all Users
Example: In this example the endpoint returns a list of all users stored on the server. Each user object contains properties such as id, name, and email.
JavaScript
//get_request.js
const axios = require('axios');
axios.get('http://localhost:3000/users')
.then(resp => {
data = resp.data;
data.forEach(e => {
console.log(`${e.first_name},
${e.last_name}, ${e.email}`);
});
})
.catch(error => {
console.log(error);
});
Run the below command to test the GET request:
node get_request.js
Output:
Ashish, Regmi, ashish@gmail.com
Anshu, abc, anshu@gmail.com
Shreya, def, shreya@gmail.com
John, aaa, John@yahoo.com
Geeks For, Geeks, gfg@gmail.com
POST Request to create a New User
Example: In this example, Send a POST request to create a new user will create a new user with the provided data.
JavaScript
//post_request.js
const axios = require('axios');
axios.post('http://localhost:3000/users', {
id: "6",
first_name: 'Geeks for',
last_name: 'Geeks',
email: 'gfg@gmail.com'
}).then(resp => {
console.log(resp.data);
}).catch(error => {
console.log(error);
});
Run the below command to test the POST Request:
node post_request.js
Output:
{
"first_name": "Geeks For",
"last_name": "Geeks",
"email": "gfg@yahoo.com",
"id": "5"
}
PUT Request to Update an Existing User
Example: In this example, send a PUT request to update an existing user this will update the user with the provided data.
JavaScript
//put_request.js
const axios = require('axios');
axios.put('http://localhost:3000/users/5/', {
first_name: 'Geeks For',
last_name: 'Geeks',
email: 'gfgofficial@gmail.com'
}).then(resp => {
console.log(resp.data);
}).catch(error => {
console.log(error);
});
Run the below command to test the PUT Request:
node put_request.js
Output:
{
first_name: 'Geeks For',
last_name: 'Geeks',
email: 'gfg@yahoo.com',
id: '5'
}
Above data is modified as
{
first_name: 'Geeks For',
last_name: 'Geeks',
email: 'gfgofficial@gmail.com',
id: '5'
}
DELETE Request to Delete a User
Example: In this example the endpoint deletes the user with the specified id from the server. After deletion, the user will no longer exist in the database.
JavaScript
//delete_request.js
const axios = require('axios');
axios.delete('http://localhost:3000/users/3/')
.then(resp => {
console.log(resp.data)
}).catch(error => {
console.log(error);
});
Run the below command to test the PUT Request:
node delete_request.js
Output:
{
id: '3',
first_name: 'Shreya',
last_name: 'def',
email: 'shreya@gmail.com'
}