Spring Boot - How to set a Request Timeout for a REST API
Last Updated :
05 Jan, 2024
In Microservice architecture, there are multiple microservices available for an application. For the application to work properly necessary services need to communicate with each other. There can be communication of messaging type or REST calls.
How does REST API Work?
In REST Calls the process is synchronous, which means suppose a service SERV1 is waiting for the response from SERV2 it will wait for an infinite time until it gets the response. Now the problem here is that if for some reason SERV2 is taking way too much time to give a response, so SERV1 keeps on waiting resulting in overall lag in the System or the Application. To overcome this type of situation we use something called Request Timed out. If Timed out time is defined as say 5 seconds, and the repose from a service didn't come in between that time it will wait no further for the response and terminate the process.
If you want to learn more about REST API visit the article on Rest API on GeeksForGeeks.
Steps to set Request Timeout for a REST API
Step 1: Create a Spring Boot Project
To create a spring boot project, go to start.spring.io, create a project with the following configurations, and add the dependencies mentioned.
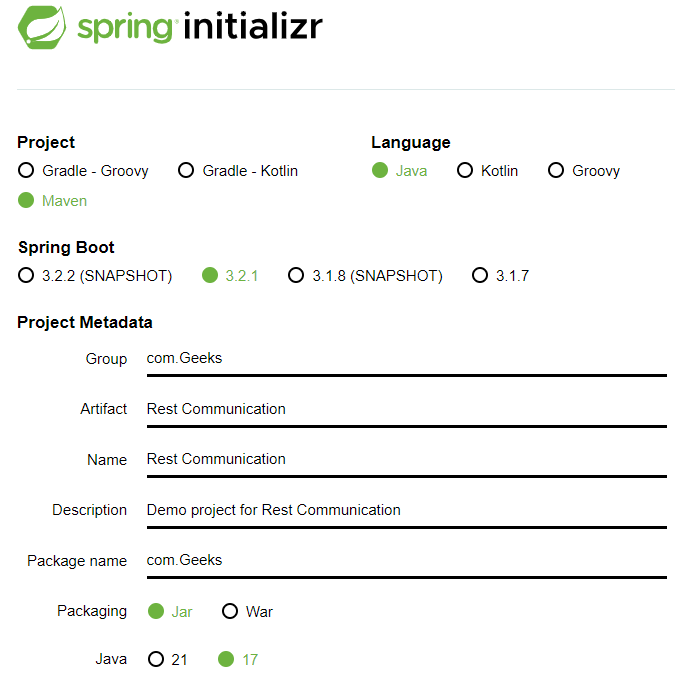
Set Project as Maven, Language as Java, Spring Boot version 3.2.1, Packaging as Jar and Java Version 17.
You can give Name and descriptions of your choice.
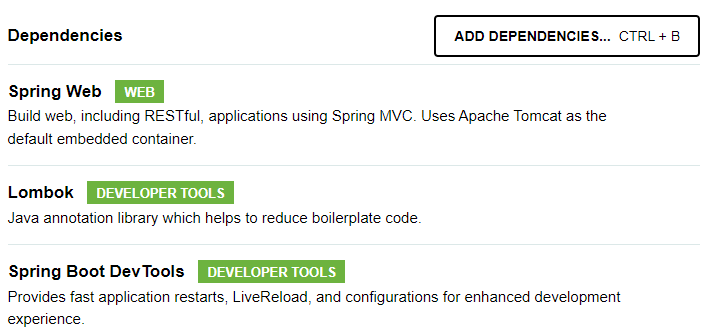
Add depencencies for
- Spring Web
- Lombok
- Spring Boot Dev Tools
Now click on Generate button there to download the project as zip file, once downloaded unzip it.
Step 2: Open the project
Use any IDE of your choice to open the project folder and wait for some time for the IDE to configure it on it's own.
Note: Here we are using Intellij IDEA IDE.
Check the folder structure below,
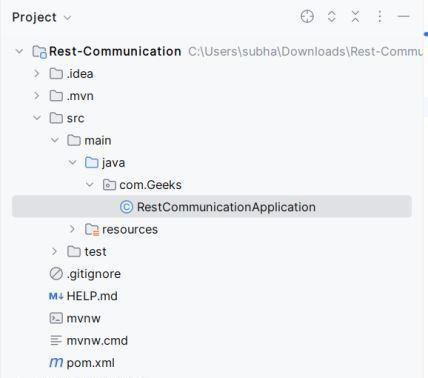
Open the pom.xml file and add the following dependency:
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
</dependency>
Check the pom.xml file below,
XML
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.2.1</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.Geeks</groupId>
<artifactId>Rest-Communication</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>Rest-Communication</name>
<description>Demo project for Rest Communication</description>
<properties>
<java.version>17</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.httpcomponents/httpclient -->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents.client5</groupId>
<artifactId>httpclient5</artifactId>
<version>5.2.3</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludes>
<exclude>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
</project>