Constructor in C++ is a special method that is invoked automatically at the time an object of a class is created. It is used to initialize the data members of new objects generally. The constructor in C++ has the same name as the class or structure. It constructs the values i.e. provides data for the object which is why it is known as a constructor.
Syntax of Constructors in C++
The prototype of the constructor looks like this:
<class-name> (){
...
}
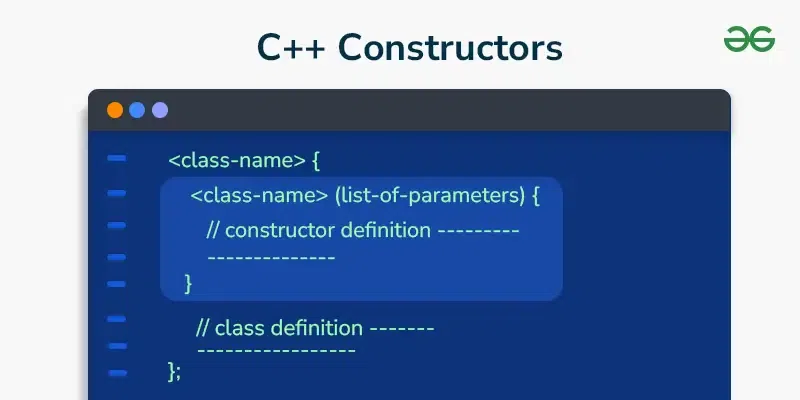
Characteristics of Constructors in C++
- The name of the constructor is the same as its class name.
- Constructors are mostly declared in the public section of the class though they can be declared in the private section of the class.
- Constructors do not return values; hence they do not have a return type.
- A constructor gets called automatically when we create the object of the class.
For a comprehensive guide on constructors and other class-related topics, explore our Complete C++ Course, which covers constructors, destructors, and other OOP features in depth.
Types of Constructor Definitions in C++
In C++, there are 2 methods by which a constructor can be declared:
1. Defining the Constructor Within the Class
<class-name> (list-of-parameters) {
// constructor definition
}
2. Defining the Constructor Outside the Class
<class-name> {
// Declaring the constructor
// Definiton will be provided outside
<class-name>();
// Defining remaining class
}
<class-name>: :<class-name>(list-of-parameters) {
// constructor definition
}
Example:
Constructor within Class
// Example to show defining
// the constructor within the class
#include <iostream>
using namespace std;
// Class definition
class student {
int rno;
char name[50];
double fee;
public:
/*
Here we will define a constructor
inside the same class for which
we are creating it.
*/
student()
{
// Constructor within the class
cout << "Enter the RollNo:";
cin >> rno;
cout << "Enter the Name:";
cin >> name;
cout << "Enter the Fee:";
cin >> fee;
}
// Function to display the data
// defined via constructor
void display()
{
cout << endl << rno << "\t" << name << "\t" << fee;
}
};
int main()
{
student s;
/*
constructor gets called automatically
as soon as the object of the class is declared
*/
s.display();
return 0;
}
Constructor outside Class
// defining the constructor outside the class
#include <iostream>
using namespace std;
class student {
int rno;
char name[50];
double fee;
public:
/*
To define a constructor outside the class,
we need to declare it within the class first.
Then we can define the implementation anywhere.
*/
student();
void display();
};
/*
Here we will define a constructor
outside the class for which
we are creating it.
*/
student::student()
{
// outside definition of constructor
cout << "Enter the RollNo:";
cin >> rno;
cout << "Enter the Name:";
cin >> name;
cout << "Enter the Fee:";
cin >> fee;
}
void student::display()
{
cout << endl << rno << "\t" << name << "\t" << fee;
}
// driver code
int main()
{
student s;
/*
constructor gets called automatically
as soon as the object of the class is declared
*/
s.display();
return 0;
}
Output:
Enter the RollNo:11
Enter the Name:Aman
Enter the Fee:10111
11 Aman 10111
Note: We can make the constructor defined outside the class as inline to make it equivalent to the in class definition. But note that inline is not an instruction to the compiler, it is only the request which compiler may or may not implement depending on the circumstances.
Constructors can be classified based on in which situations they are being used. There are 4 types of constructors in C++:
- Default Constructor: No parameters. They are used to create an object with default values.
- Parameterized Constructor: Takes parameters. Used to create an object with specific initial values.
- Copy Constructor: Takes a reference to another object of the same class. Used to create a copy of an object.
- Move Constructor: Takes an rvalue reference to another object. Transfers resources from a temporary object.
A default constructor is a constructor that doesn’t take any argument. It has no parameters. It is also called a zero-argument constructor.
Syntax of Default Constructor
className() {
// body_of_constructor
}
The compiler automatically creates an implicit default constructor if the programmer does not define one.
Parameterized constructors make it possible to pass arguments to constructors. Typically, these arguments help initialize an object when it is created. To create a parameterized constructor, simply add parameters to it the way you would to any other function. When you define the constructor’s body, use the parameters to initialize the object.
Syntax of Parameterized Constructor
className (parameters...) {
// body
}
If we want to initialize the data members, we can also use the initializer list as shown:
MyClass::MyClass(int val) : memberVar(val) {};
A copy constructor is a member function that initializes an object using another object of the same class.
Syntax of Copy Constructor
Copy constructor takes a reference to an object of the same class as an argument.
ClassName (ClassName &obj)
{
// body_containing_logic
}
Just like the default constructor, the C++ compiler also provides an implicit copy constructor if the explicit copy constructor definition is not present.
Here, it is to be noted that, unlike the default constructor where the presence of any type of explicit constructor results in the deletion of the implicit default constructor, the implicit copy constructor will always be created by the compiler if there is no explicit copy constructor or explicit move constructor is present.
The move constructor is a recent addition to the family of constructors in C++. It is like a copy constructor that constructs the object from the already existing objects., but instead of copying the object in the new memory, it makes use of move semantics to transfer the ownership of the already created object to the new object without creating extra copies.
It can be seen as stealing the resources from other objects.
Syntax of Move Constructor
className (className&& obj) {
// body of the constructor
}
The move constructor takes the rvalue reference of the object of the same class and transfers the ownership of this object to the newly created object.
Like a copy constructor, the compiler will create a move constructor for each class that does not have any explicit move constructor.
Conclusion
In summary, constructors in C++ are special methods that initialize objects of a class. They can be default (with no arguments), parameterized (with specific values), copy constructors (for deep copying), or move constructors (for resource transfer). Understanding these types and their usage is essential for effective object initialization and management.
If you are confused about what you should study next on this topic, refer to the article – A Comprehensive Guide to Constructors in C++: Everything You Need to Know
Frequently Asked Questions on C++ Constructors
What Are the Functions That Are Generated by the Compiler by Default, If We Do Not Provide Them Explicitly?
The functions that are generated by the compiler by default if we do not provide them explicitly are:
- Default Constructor
- Copy Constructor
- Move Constructors
- Assignment Operator
- Destructor
Can We Make the Constructors Private?
Yes, in C++, constructors can be made private. This means that no external code can directly create an object of that class.
How Constructors Are Different from a Normal Member Function?
A constructor is different from normal functions in following ways:
- Constructor has same name as the class itself
- Default Constructors don’t have input argument however, Copy and Parameterized Constructors have input arguments
- Constructors don’t have return type
- A constructor is automatically called when an object is created.
- It must be placed in public section of class.
- If we do not specify a constructor, C++ compiler generates a default constructor for object (expects no parameters and has an empty body).
Can We Have More Than One Constructor in a Class?
Yes, we can have more than one constructor in a class. It is called Constructor Overloading.
Related Articles: